flutter 本地存储和 Android中的数据库中是怎样实现的
时间:2024-02-17 06:39:32 编辑:勤于奋老勤
如何使用 Shared Preferences?
在 Android 中,你可以使用 SharedPreferences API 来存储少量的键值对。
在 Flutter 中,使用 Shared_Preferences 插件 实现此功能。这个插件同时包装了 Shared Preferences 和 NSUserDefaults(iOS 平台对应 API)的功能。
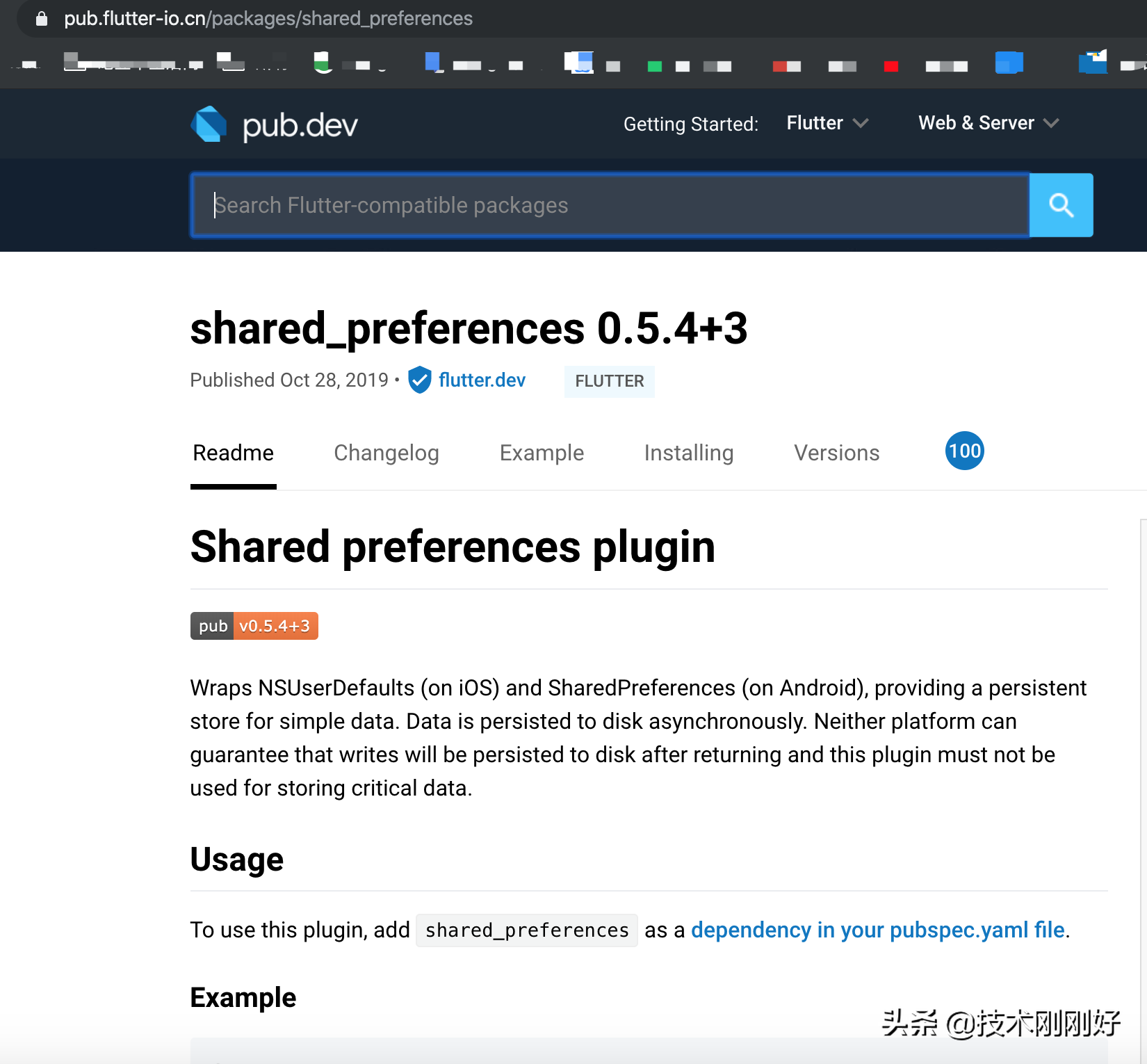
用法
要使用此插件,请在pubspec.yaml文件中添加shared_preferences为依赖项。
import 'package:flutter/material.dart'; import 'package:shared_preferences/shared_preferences.dart'; void main() { runApp(MaterialApp( home: Scaffold( body: Center( child: RaisedButton( onPressed: _incrementCounter, child: Text('Increment Counter'), ), ), ), )); } _incrementCounter() async { SharedPreferences prefs = await SharedPreferences.getInstance(); int counter = (prefs.getInt('counter') ?? 0) + 1; print('Pressed $counter times.'); await prefs.setInt('counter', counter); }
测试
您可以SharedPreferences通过运行以下代码在测试中填充初始值:
const MethodChannel('plugins.flutter.io/shared_preferences') .setMockMethodCallHandler((MethodCall methodCall) async { if (methodCall.method == 'getAll') { return {}; // set initial values here if desired } return null; });
在 Flutter 中如何使用 SQLite?
在 Android 中,你会使用 SQLite 来存储可以通过 SQL 进行查询的结构化数据。
在 Flutter 中,使用 SQFlite 插件实现此功能。
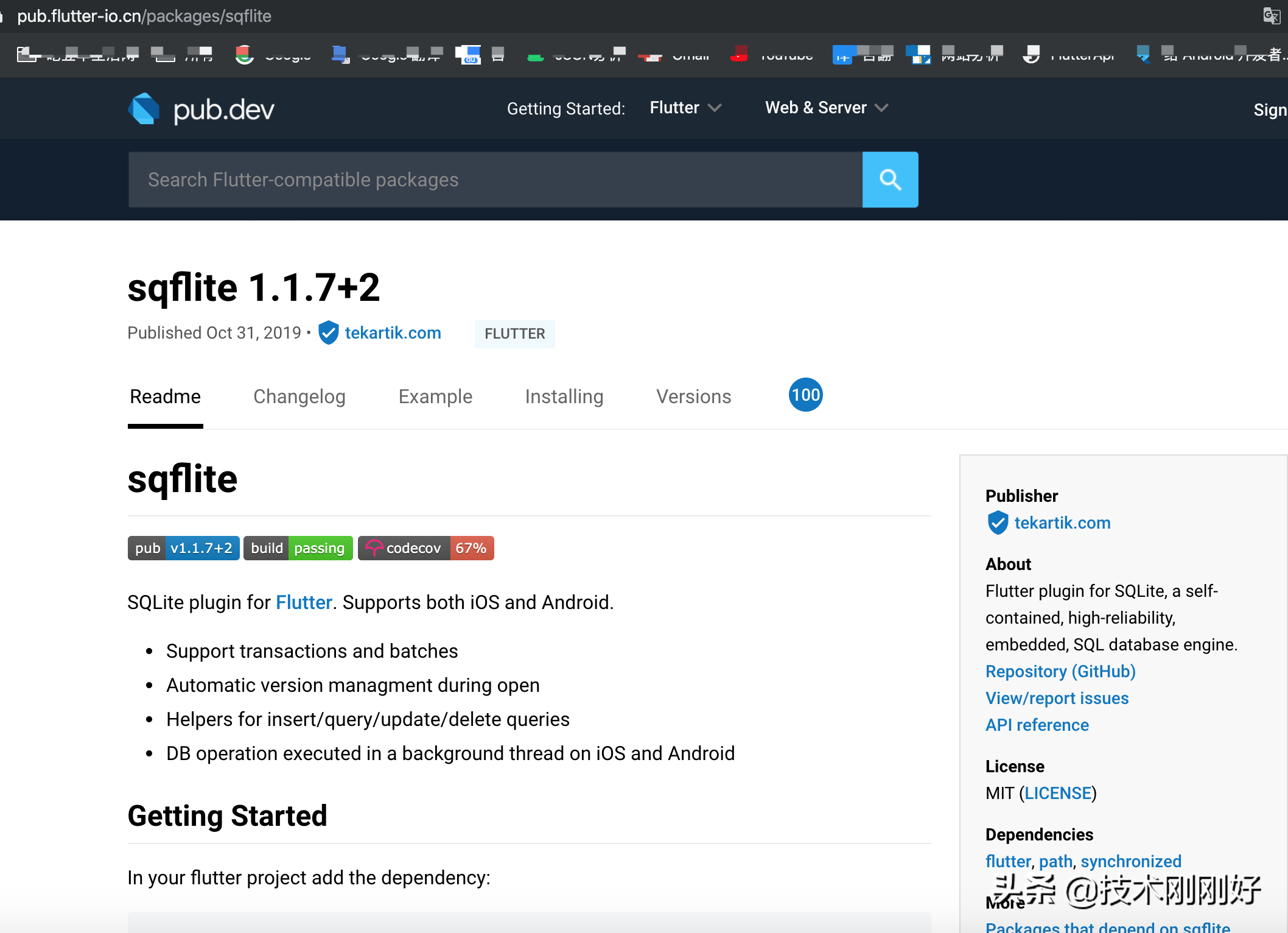
Flutter的 SQLite插件。同时支持iOS和Android。
- 支持交易和批次
- 打开期间自动版本管理
- 插入/查询/更新/删除查询的助手
- 在iOS和Android的后台线程中执行的数据库操作
入门
在flutter项目中添加依赖项:
dependencies: ... sqflite: ^1.1.7+1
用法示例
进口 sqflite.dart
打开数据库
SQLite数据库是文件系统中由路径标识的文件。如果是相对路径,则该路径相对于所获得的路径,该路径是getDatabasesPath()Android上的默认数据库目录和iOS上的documents目录。
var db = await openDatabase('my_db.db');
有一个基本的迁移机制可以处理打开期间的模式更改。
许多应用程序使用一个数据库,并且永远不需要关闭它(当应用程序终止时,它将关闭)。如果要释放资源,可以关闭数据库。
await db.close();
原始的SQL查询
演示代码执行原始SQL查询
// Get a location using getDatabasesPath var databasesPath = await getDatabasesPath(); String path = join(databasesPath, 'demo.db'); // Delete the database await deleteDatabase(path); // open the database Database database = await openDatabase(path, version: 1, onCreate: (Database db, int version) async { // When creating the db, create the table await db.execute( 'CREATE TABLE Test (id INTEGER PRIMARY KEY, name TEXT, value INTEGER, num REAL)'); }); // Insert some records in a transaction await database.transaction((txn) async { int id1 = await txn.rawInsert( 'INSERT INTO Test(name, value, num) VALUES("some name", 1234, 456.789)'); print('inserted1: $id1'); int id2 = await txn.rawInsert( 'INSERT INTO Test(name, value, num) VALUES(?, ?, ?)', ['another name', 12345678, 3.1416]); print('inserted2: $id2'); }); // Update some record int count = await database.rawUpdate( 'UPDATE Test SET name = ?, VALUE = ? WHERE name = ?', ['updated name', '9876', 'some name']); print('updated: $count'); // Get the records List
SQL助手
使用助手的示例
final String tableTodo = 'todo'; final String columnId = '_id'; final String columnTitle = 'title'; final String columnDone = 'done'; class Todo { int id; String title; bool done; Map toMap() { var map = { columnTitle: title, columnDone: done == true ? 1 : 0 }; if (id != null) { map[columnId] = id; } return map; } Todo(); Todo.fromMap(Map map) { id = map[columnId]; title = map[columnTitle]; done = map[columnDone] == 1; } } class TodoProvider { Database db; Future open(String path) async { db = await openDatabase(path, version: 1, onCreate: (Database db, int version) async { await db.execute(''' create table $tableTodo ( $columnId integer primary key autoincrement, $columnTitle text not null, $columnDone integer not null) '''); }); } Future insert(Todo todo) async { todo.id = await db.insert(tableTodo, todo.toMap()); return todo; } Future getTodo(int id) async { Listmaps = await db.query(tableTodo, columns: [columnId, columnDone, columnTitle], where: '$columnId = ?', whereArgs: [id]); if (maps.length > 0) { return Todo.fromMap(maps.first); } return null; } Future delete(int id) async { return await db.delete(tableTodo, where: '$columnId = ?', whereArgs: [id]); } Future update(Todo todo) async { return await db.update(tableTodo, todo.toMap(), where: '$columnId = ?', whereArgs: [todo.id]); } Future close() async => db.close(); }
读取结果
假设有以下读取结果:
生成的地图项为只读
// get the first record Map mapRead = records.first; // Update it in memory...this will throw an exception mapRead['my_column'] = 1; // Crash... `mapRead` is read-only
如果要在内存中进行修改,则需要创建一个新地图:
// get the first record Map map = Map.from(mapRead); // Update it in memory now map['my_column'] = 1;
相关文章
-
近日,有我爱卡论坛的网友发帖称,自己信用卡的额度原来是20000,在冷冻3个月后,接到短信提醒说额度调整为2000元。对此,网友大呼,这种冷冻提额实在“太坑爹”了。据了解,原来这位网友想尝试一下“传说中”的交行冷冻提额,便打客服电话咨询,客服自称这个系统每个月会审核用户的用卡情况,进行调整,说是对长[详细]
-
问题一:万一得了大病怎么办?在人的一生中,有两个我们无法预知的突发事件:疾病和意外。如果一个人真的遭遇大病或残疾,有三笔费用是必须要面对的:医疗费、康复费、收入损失费。社保可以为你解决基本医疗费的问题,但如果得了重病,需要进口药或者复杂的手术,那就只能自费了。这是一笔巨大的开支,特别是病后的恢复。医[详细]
-
近日,吴起县人民法院成功调解了一起借贷担保纠纷。 2011年5月24日,原告张某某为好友陈某某在被告某银行处借款提供了个人担保。该笔借款期限从2011年5月24日至2014年5月22日。张某某提供担保的保证期限为借款到期之日起两年内,即从2014年5月22日至2016年5月22日。借款到期后陈[详细]
-
装修大计,水电先行,电路作为隐蔽工程,一旦发生事故,轻则全屋短路,重则引发事故。可见做好电线布局十分重要,电线不能直接埋进墙体或者地面,应该进行穿线,防止电线绝缘层受损。因此,一款好的电工套管显得十分重要。然而,市面上的电工套管琳琅满目,如何选择一款性价比高、质量过硬的电工套管成了摆在业主面前的一道[详细]
-
爱养花的朋友来自各个领域,商人也是其中之一。大部分花店都为很多商务场所和家庭提供了各种花卉盆栽。他们对花卉的挑选和摆放都有很高的要求。今天这几种很受商业人士喜爱的花,不仅好看还有好寓意!白掌白掌,也被称为“一帆风顺”,代表着万事顺利。它四季常青,不会掉叶,适合在酸性泥炭土中生长,喜欢半阴半阳的环境,[详细]